Add monetization to your Trello Power-Up
You can configure how your Trello Power-Up is monetized on Optro and there are two types of subscription models available:
Traditional - the entire Power-Up requires a subscription (e.g. users will not be able to use the Power-Up without a paid subscription)
Freemium - certain features require a subscription (e.g. users can access use some of the Power-Ups features for free, but for more advanced features they will require a paid subscription)
If you choose to use the Freemium model, you can customize which features are paid versus free using a paywall. You can also use a License Status indicator (e.g. “You are on the Free Plan”) to display a user’s license status within the Power-Up and upsell the more premium features. See example below.
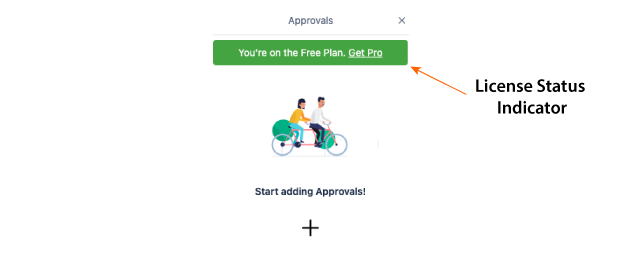
To enable monetization in your Power-Up use the Optro React UI in collaboration with the Optro API Client. These libraries provide everything you need to monetize your Power-Up and customize which features are paid versus free. Follow the steps below to add monetization to your Power-Up.
Before adding monetization, please ensure you have registered with Optro Vendor.
1. Ensure you have set up the Trello API Provider
Make sure you’ve set up the Trello API Provider first and ensure that it is above the LicenseProvider in the React hierarchy.
2. Provide access to the Optro License status
First of all, add the LicenseProvider higher-order component to the root of your React project (e.g. router):
import {LicenseProvider, TrelloProvider} from '@optro/ui-react';
import {OptroLicenseApi} from '@optro/api-client';
// Create a caching Optro License API client
const optroClient = new OptroLicenseApi(
OPTRO_API_KEY,
POWERUP_ID
);
function ReactRoot() {
return (
<LicenseProvider
apiKey={OPTRO_API_KEY} // get it from vendor.optro.cloud
powerupId={POWERUP_ID} // get it from trello.com/power-ups/admin
optroClient={optroClient} // Optro API Client (see above)
licenseType={'board'} // License by 'board' or 'member' (less common)
>
...
</LicenseProvider>
);
}
You can then access the license status of the board or member using the useLicense hook in any component further down the hierarchy:
import {useLicense} from '@optro/ui-react';
function ReactComponent() {
const lic = useLicense();
if(lic.licensed) {
// we have a licensed user
}
}
3. Conditionally Allow/Deny Features (Paywalling)
If you are making certain features subscription-only, you may want to hide, or render alternative content when the user does not have a valid subscription.
Using the LicenseConditional component, you can display different content based on the LicenseProvider data:
<LicenseConditional
unlicensed={<div>This is rendered when the user is not licensed</div>}
>
This is rendered when the user has a valid subscription
</LicenseConditional>
4. Display Licence Status
This component helps you indicate what level of subscription a customer has and provide an easy way for users to start a subscription, or manage their existing subscription.
LicenseStatus shows on screen the status of the current board or member license with a link to subscribe or manage their existing subscription:
<LicenseStatus
isPro={lic.licensed} // You can get this boolean from the useLicense() hook
onGetPro={functionToCallOnUpsell} // You can call a function when the user wants to upgrade (e.g. direct to Optro Listing page)
powerupId={yourPowerupId}
locale={one of "en" | "de" | "fr" | "es"} // Trello provides window.locale for this
/>
Next Steps
Now that the License API’s have been configured correctly, you will need to setup license checking within the Optro API client to ensure it manages the license status of Trello users correctly.