Use the Trello API with React
Your Power-Up will need to interact with the Trello API to perform some operations. For example:
Get information on Trello data, such as Cards and Boards and their metadata
Set metadata on Trello Cards and Boards
Creating, updating and deleting Cards
Use this guide to find out how to use the Trello API Provider and associated React Hook to access the Trello API, also known as t
.
1. Install the @optro/api-client package
Before you can use the Optro UI, you need to install it:
# Using Yarn
yarn add @optro/api-client
# Using NPM
npm install @optro/api-client
2. Add the TrelloProvider to the root of your React project
Find out how to access the Trello API throughout your Power-Up using the TrelloProvider at the root level and then calling the useTrelloApi hook to access the client.
First, place the following code at the root of your project:
import {TrelloProvider} from '@optro/ui-react';
const t = window.TrelloPowerUp.iframe();
function ReactRoot() {
return (
<TrelloProvider t={t}>
...
</TrelloProvider>
);
}
3. Use the useProvidedTrello() React Hook to use the Trello API
Next, use the following React Hook which provides access to the Trello API in a component that is lower down the hierarchy:
import {useProvidedTrello} from '@optro/ui-react';
function ReactComponent() {
const t = useProvidedTrello();
// Use the Trello API
t.showCard(item.card.id);
}
4. Use TypeScript Code Completion
As the Trello types are in the types/trello.d.ts
file in the template and any generated projects, you can use these TypeScript types to enable auto-completion on the Trello JavaScript API.
If you’re not using the generator or template, you can use the types in your own project using the Trello Types File.
Check out an example of this auto-completion in IntelliJ IDEA:
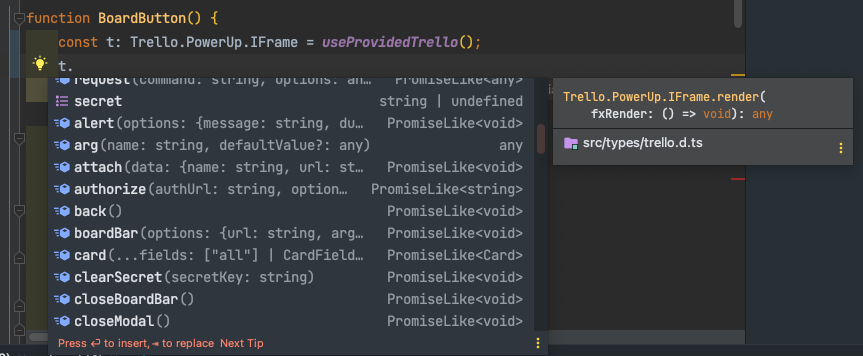
This form of autocompletion is supported in most IDE’s e.g. VS Code, Atom, WebStorm.
API Reference
If you want full documentation on what functions are available in the Trello API, check out these guides from Atlassian.
Next Steps
You should now have access to the Trello API inside your React components, provided they have a parent component that contains the provider.
Next, let’s show you how capabilities are handled in the Power-Up and how you can extend them.